์ธํ๋ฐ ๊น์ํ๋์ ์คํ๋ง MVC 1ํธ ๊ฐ์ ๋ด์ฉ์ ๋ฐํ์ผ๋ก ์์ฑํ ๊ธ์ ๋๋ค.
1. ์๋ธ๋ฆฟ ๋ฑ๋กํ๊ธฐ
package hello.servlet;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.servlet.ServletComponentScan;
@ServletComponentScan // ์๋ธ๋ฆฟ ์๋ ๋ฑ๋ก
@SpringBootApplication
public class ServletApplication {
public static void main(String[] args) {
SpringApplication.run(ServletApplication.class, args);
}
}
- @ServletComponentScan: ์คํ๋ง ๋ถํธ์์ ์๋ธ๋ฆฟ์ ์ง์ ๋ฑ๋กํด์ ์ฌ์ฉํ ์ ์๋๋ก ์ง์ํ๋ ์ด๋ ธํ ์ด์
@WebServlet(name = "exampleServlet", urlPatterns = "/example")
public class ExampleServlet extends HttpServlet {
@Override
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("ExampleServlet.service");
System.out.println("request = " + request);
System.out.println("response = " + response);
}
}
- @WebServlet: ํด๋น ํด๋์ค๋ฅผ ์๋ธ๋ฆฟ์ผ๋ก ๋ฑ๋กํ๋ ์ด๋ ธํ ์ด์
- name: ์๋ธ๋ฆฟ์ ์ด๋ฆ์ ์ค์ ํ ์ ์์
- urlPatterns: ์๋ธ๋ฆฟ๊ณผ ๋งคํํ URL์ ์ค์ ํ ์ ์์
- ์๋ธ๋ฆฟ๊ณผ ๋งคํ๋ URL์ด ํธ์ถ๋๋ฉด, ์๋ธ๋ฆฟ ์ปจํ
์ด๋๋ ํด๋น ์๋ธ๋ฆฟ์ service()
๋ฉ์๋๋ฅผ ์คํ
- ์์ ๊ฒฝ์ฐ๋ /example
URL๋ก ์์ฒญ์ด ์ค๋ฉด, ExampleServlet
์ service()
๊ฐ ํธ์ถ๋๋ค.
- ๋ธ๋ผ์ฐ์ ์ฃผ์์ฐฝ์ localhost:8080/example
์ ์
๋ ฅํ ๋ค ์ฝ์์ฐฝ์ ํ์ธํด๋ณด์.
2. HttpServletRequest
@Override
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
printRequestInfo(request);
}
private void printRequestInfo(HttpServletRequest request) {
System.out.println("[START LINE ์กฐํ]");
System.out.println("HTTP ๋ฉ์๋ ์ ๋ณด = " + request.getMethod());
System.out.println("์์ฒญ URL ์ ๋ณด = " + request.getRequestURL());
System.out.println();
System.out.println("[ํค๋ ์ ๋ณด ์กฐํ]");
request.getHeaderNames().asIterator()
.forEachRemaining(headerName -> System.out.println(headerName + " = " + request.getHeader(headerName)));
System.out.println("์ปจํ
์ธ ํ์
์ ๋ณด = " + request.getContentType());
}
- HttpServletRequest: HTTP ์์ฒญ ๋ฉ์ธ์ง๋ฅผ ํ์ฑํ ๊ฒฐ๊ณผ๊ฐ ๋ด๊ธฐ๋ ํด๋์ค
- ๊ฐ๋ฐ์๋ HttpServletRequest
๋ฅผ ํตํด HTTP ์์ฒญ ๋ฉ์ธ์ง๋ฅผ ํธ๋ฆฌํ๊ฒ ์ฌ์ฉํ ์ ์๋ค.
- HttpServletRequest๊ฐ ์ ๊ณตํ๋ ๊ธฐ๋ฅ
- START LINE ์กฐํ
- HTTP ๋ฉ์๋ ์ ๋ณด: request.getMethod()
- ์์ฒญURL: request.getRequestURI()
- ๊ทธ ์ธ ์ฟผ๋ฆฌ ์คํธ๋ง, ์คํค๋ง, ํ๋กํ ์ฝ ์ ๋ณด ๋ฑ ์กฐํ ๊ฐ๋ฅ
- ํค๋ ์กฐํ
- ๋ชจ๋ ํค๋ ์ ๋ณด ์กฐํ: request.getHeaderNames()
- ํน์ ํค๋ ์ ๋ณด๋ง ์กฐํ: request.getHeader(headerName)
- HTTP ์์ฒญ ๋ฉ์ธ์ง์ ์ปจํ ์ธ ํ์ ์กฐํ: request.getContentType()
- ๊ทธ ์ธ ๋ค์ํ ํค๋ ์ ๋ณด ์กฐํ ๊ฐ๋ฅ
- ๋ฐ๋ ์กฐํ: form ํ๋ผ๋ฏธํฐ ํ์ ์กฐํ, ๋ฉ์ธ์ง ๋ฐ๋ ๋ฐ์ดํฐ ์ง์ ์กฐํ ๊ฐ๋ฅ
- ์์ ์ ์ฅ์ ๊ธฐ๋ฅ
- ์ ์ฅ: request.setAttribute(name, value)
- ์กฐํ: request.getAttribute(name)
- ์ธ์ ๊ด๋ฆฌ ๊ธฐ๋ฅ
- request.getSession(create: true)
์คํ ๊ฒฐ๊ณผ
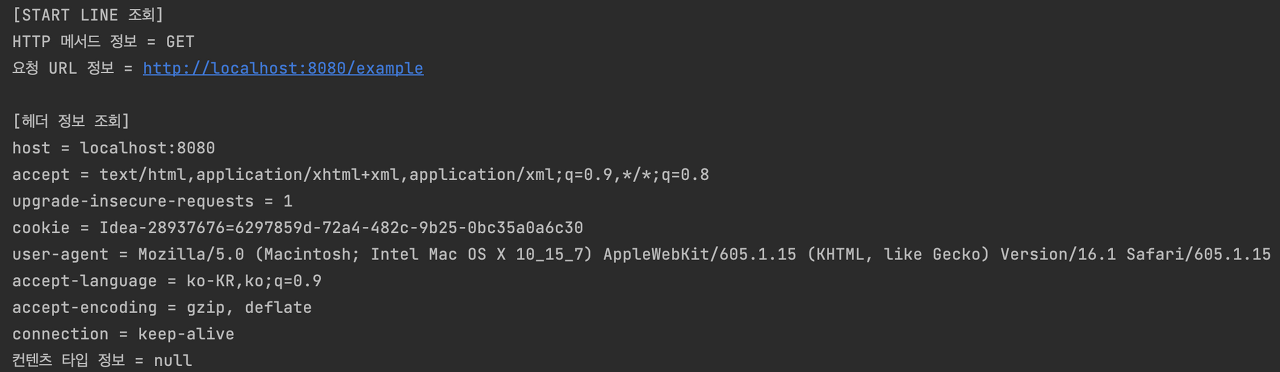
3. HTTP ์์ฒญ ๋ฐ์ดํฐ ์ ์ก
HTTP ์์ฒญ ๋ฉ์ธ์ง๋ฅผ ํตํด ํด๋ผ์ด์ธํธ์์ ์๋ฒ๋ก ๋ฐ์ดํฐ๋ฅผ ์ ์กํ๋ ๋ฐฉ๋ฒ์ ํฌ๊ฒ 3๊ฐ์ง๊ฐ ์๋ค.
(1) GET - ์ฟผ๋ฆฌ ํ๋ผ๋ฏธํฐ ์ด์ฉ
@Override
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("username = " + request.getParameter("username"));
System.out.println("age = " + request.getParameter("age"));
}
- ๋ฉ์ธ์ง ๋ฐ๋ ์์ด URL์ ์ฟผ๋ฆฌ ํ๋ผ๋ฏธํฐ๋ฅผ ์ฌ์ฉํด์ ๋ฐ์ดํฐ ์ ๋ฌ
- ๊ทธ๋๋ก ๋ ธ์ถ๋๋ ์์ญ์ด๋ฏ๋ก, ์ธ๋ถ์ ๋ณด์ฌ์ ธ๋ ์๊ด์๋ ๋ฐ์ดํฐ๋ฅผ ์ ๋ฌํ ๋ ์ฌ์ฉ
- ?
๋ก ์์, ๊ฐ ํ๋ผ๋ฏธํฐ๋ค์ &
๋ก ๊ตฌ๋ถ
- ์คํ: http://localhost:8080/example?username=kim&age=20
(2) POST - HTML Form ์ด์ฉ
- HTML Form์ ํตํด ๋ฐ์ดํฐ๋ฅผ ์ ์กํ๋ ๋ฐฉ๋ฒ
- content-type์ด application/x-www-form-urlencoded
๊ฐ ๋จ
- ๋ฉ์ธ์ง ๋ฐ๋์ ์ฟผ๋ฆฌ ํ๋ผ๋ฏธํฐ ํ์์ผ๋ก ๋ฐ์ดํฐ๊ฐ ๋ค์ด๊ฐ
ex) username=kim&age=20
- ํ์์ด ๋์ผํ๋ฏ๋ก ์ฟผ๋ฆฌ ํ๋ผ๋ฏธํฐ ์กฐํ ๋ฉ์๋์ ๊ฐ์ ๋ฐฉ๋ฒ์ผ๋ก ์กฐํ ๊ฐ๋ฅ
๐ก ์ฟผ๋ฆฌ ํ๋ผ๋ฏธํฐ ํ์์ผ๋ก ๋ฐ์ดํฐ๋ฅผ ์ ์กํ ๋๋, HTTP ๋ฉ์ธ์ง ๋ฐ๋์ ๋ฐ์ดํฐ๊ฐ ๋ค์ด๊ฐ์ง ์๊ธฐ ๋๋ฌธ์ content-type์ด null์ด์๋ค. ํ์ง๋ง HTML Form์ ์ด์ฉํด ๋ฐ์ดํฐ๋ฅผ ์ ์กํ๋ฉด, HTTP ๋ฉ์ธ์ง ๋ฐ๋์ ๋ฐ์ดํฐ๊ฐ ๋ค์ด๊ฐ๊ธฐ ๋๋ฌธ์ content-type์ด application/x-www-form-urlencoded๊ฐ ๋๋ค.
ํฌ์คํธ๋งจ์ ์ด์ฉํด ๊ฐ๋จํ๊ฒ ํ ์คํธํด๋ณด์.
์ฝ์์ฐฝ์ ํ์ธํ๋ฉด ์คํ ๊ฒฐ๊ณผ๊ฐ ์ ๋๋ก ์ฐํ๋ ๊ฒ์ ํ์ธํ ์ ์๋ค.
(3) HTTP ๋ฉ์ธ์ง ๋ฐ๋์ ์ง์ ๋ด์ ์ ์ก
- HTTP ๋ฉ์ธ์ง ๋ฐ๋์ ๋ฐ์ดํฐ๋ฅผ ์ง์ ๋ด์์ ์ ์กํ๋ ๋ฐฉ๋ฒ
- HTTP API์์ ์ฃผ๋ก ์ฌ์ฉํ๋ ๋ฐฉ์
- ๋ฐ์ดํฐ ํ์์ ์ฃผ๋ก JSON ์ฌ์ฉ
@Override
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
ServletInputStream inputStream = request.getInputStream();
String data = StreamUtils.copyToString(inputStream, StandardCharsets.UTF_8);
System.out.println("message body = " + data);
response.getWriter().write("ok!");
}
- ํ ์คํธ ํ์ ๋ฐ์ดํฐ ์ ์ก
- content-type: text/plain
- ๋ฉ์ธ์ง ๋ฐ๋์ ๋ด๊ธฐ๋ ๋ด์ฉ: message ์ ์ก ํ
์คํธ
- request.getInputStream(): HTTP ๋ฉ์ธ์ง ๋ฐ๋ ๋ด์ฉ์ ์คํธ๋ฆผ์ผ๋ก ๊บผ๋ด์ด
- inputStream
์ byte์ฝ๋๋ฅผ ๋ฐํํ๋ฏ๋ก, ์ฐ๋ฆฌ๊ฐ ์ฝ์ ์ ์๋ ๋ฌธ์๋ก ๋ณํํ๊ธฐ ์ํด ์ธ์ฝ๋ฉ ํ์์ UTF-8๋ก ์ง์
ํฌ์คํธ๋งจ์ ์ด์ฉํด ๊ฐ๋จํ๊ฒ ํ ์คํธํด๋ณด์.
- JSON ํ์ ๋ฐ์ดํฐ ์ ์ก
- HTTP API์์ ์ฃผ๋ก ์ฌ์ฉํ๋ ๋ฐฉ์์ด๋ค.
- content-type: application/json
- ๋ฉ์ธ์ง ๋ฐ๋์ ๋ด๊ธฐ๋ ๋ด์ฉ: {"username": "kim", "age": 20}
ํ
์คํธ ์งํ ์ , ๋ค์ด์ค๋ JSON ํ์์ ๋ฐ์ดํฐ๋ฅผ ๊ฐ์ฒด๋ก ๋ณํํ ์ ์๋๋ก ๋์ผ ํจํค์ง์ ExampleData
ํด๋์ค๋ฅผ ํ๋ ์ถ๊ฐํ๋ค.
@Getter
@Setter
public class ExampleData {
private String username;
private int age;
}
JSON ๋ฐ์ดํฐ๋ฅผ ๊ฐ์ฒด๋ก ํ์ฑํ๋ ค๋ฉด, JSON ๋ณํ ๋ผ์ด๋ธ๋ฌ๋ฆฌ๋ฅผ ์ถ๊ฐํด์ผ ํ๋ค. ์ฌ๊ธฐ์๋ Jackson ๋ผ์ด๋ธ๋ฌ๋ฆฌ์ ObjectMapper
๋ฅผ ์ด์ฉํ์ฌ ํ์ฑํ์๋ค.
@WebServlet(name = "exampleServlet", urlPatterns = "/example")
public class ExampleServlet extends HttpServlet {
private ObjectMapper objectMapper = new ObjectMapper();
@Override
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
ServletInputStream inputStream = request.getInputStream();
String data = StreamUtils.copyToString(inputStream, StandardCharsets.UTF_8);
System.out.println("message body = " + data);
ExampleData exampleData = objectMapper.readValue(data, ExampleData.class);
System.out.println("exampleData.username = " + exampleData.getUsername());
System.out.println("exampleData.age = " + exampleData.getAge());
response.getWriter().write("ok!");
}
์ด์ ํฌ์คํธ๋งจ์ ์ด์ฉํด ๊ฐ๋จํ๊ฒ ํ ์คํธํด๋ณด์.
์ ์์ ์ผ๋ก ์คํ๋๋ ๊ฒ์ ํ์ธํ ์ ์๋ค.
'๐๐ฉ๐ซ๐ข๐ง๐ > ๐๐๐ (๐)' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
HttpServletResponse ๋ฐํํ๊ธฐ (1) | 2023.11.08 |
---|---|
์๋ธ๋ฆฟ์ด๋? (0) | 2023.10.17 |